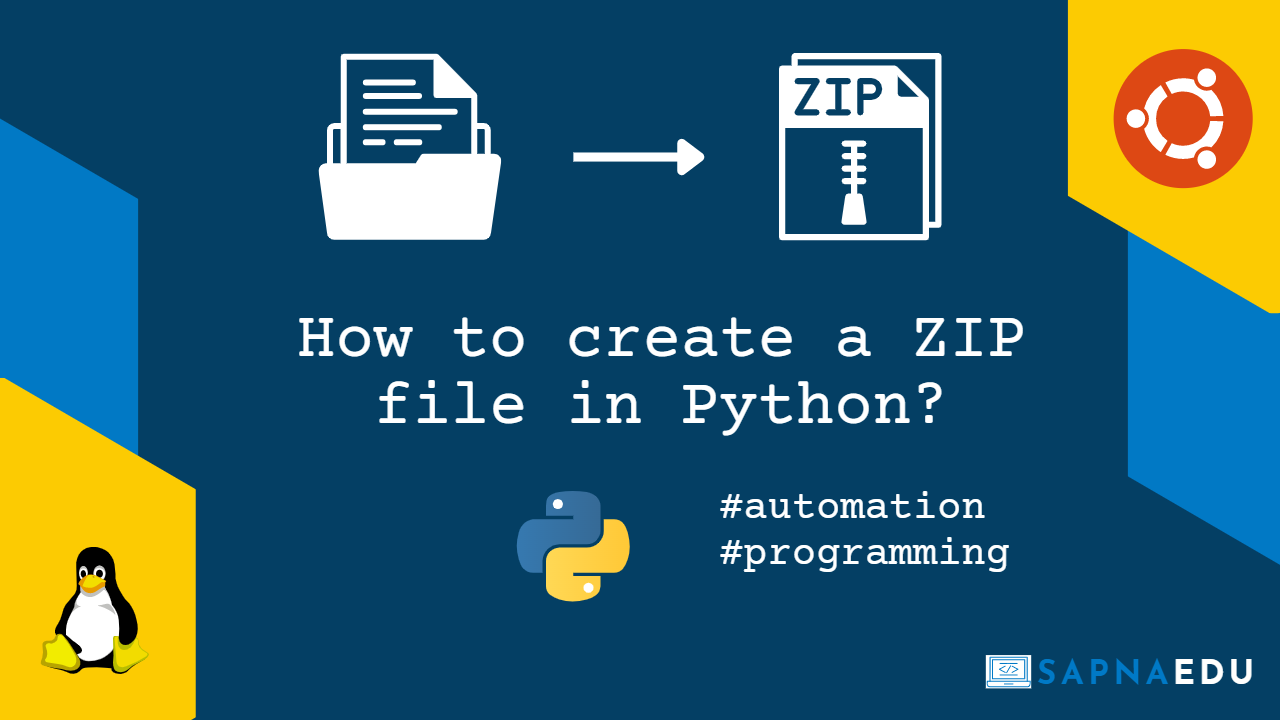
How to create a zip file in Python
Continuing on our previous topics on automation in Python, let us look at a very common task of creating a zip file from a folder. At the end of this article, you will learn how to create a Zip file in Python.
We often come across multiple scenarios, where we need to send multiple files via your web-application. Instead of sending separate files, we could all zip it together and send it as a single zip file. In this article, we will specifically look at 2 scenarios:
- Create a zip file from a given input folder which consists of both files and folders
- Unzip the given zip file and extract all the files.
Create Zip file using shutil
The easiest way of creating a zip in Python is using shutil
package. So you specify the input directory and you create a zip file consists of both files and folders. You just need to specify the input directory that you wish to zip and the output filename to create a zip file. Please refer to the code snippet below:
import shutil
import os
#-------------------------------------------------------
# Create zip file from the list of all files in
# specified directory
#-------------------------------------------------------
def create_zip_file_v2(directory:str)->str:
zip_file = f"{os.getcwd()}/output/test"
zip_path = shutil.make_archive(zip_file, 'zip', directory) #This create test.zip in output folder
return zip_path
Create Zip file using zipfile
When we are generating a zip file dynamically, the most practical option would be to use zipfile
package. It allows us to add the files while creating a zip file rather than the complete directory. Please refer to the code snippet below:
import os
import zipfile
#------------------------------------------------------------
# Create a zip file from the list of all the files
#------------------------------------------------------------
def create_zip_file(file_list:list)->str:
zip_file = f"{os.getcwd()}/output/test.zip"
with zipfile.ZipFile(zip_file, "w") as zf:
for file in file_list:
zf.write(file, os.path.basename(file))
return zip_file
Unzip the Zip file
Now that, we have created the zipfile, let us look at extracting all the files given a zip file. We need to use extractall()
method, which will extract all the contents from the zip file to the output directory. If the output directory path is not specified, it will extract to the current directory. Please refer to the code snippet below:
import os
import zipfile
#-------------------------------------------------#
# Unzip the file #
#-------------------------------------------------#
def unzip_file(zip_file:str)->str:
output_folder = f"{os.getcwd()}/output/test1"
with zipfile.ZipFile(zip_file, 'r') as zip_ref:
zip_ref.extractall(output_folder)
return output_folder
I hope you find this article helpful. The complete code to create a zip file can be downloaded from my GitHub account here: https://github.com/kirancshet/Create-Zip-Python
As an exercise, can you explore the following:
- Create a zip file of all the images in the input folder
- Send an email with the zip file as an attachment. Please refer to my article here: How to send email from your Gmail account with multiple attachments in Python?
- Send an SMS indicating the report has been sent. Please refer to my article here: How to send SMS via Twilio in Python?
558 total views, 2 views today