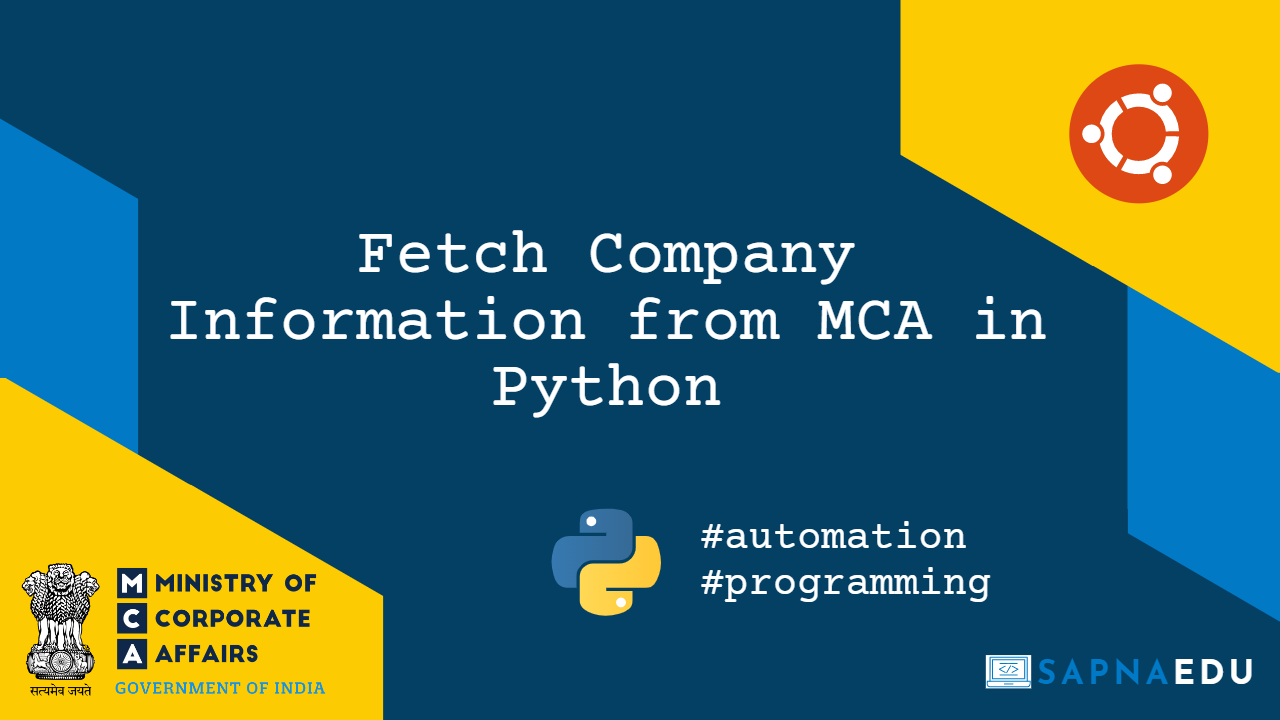
In this article, we will explore how to fetch Company Information from MCA in Python. This is part of a series of article which I intend to cover on automating boring stuff.
This article is first in the series of articles, where we explore automating MCA website typically used to obtain information like Director details, Company information, Signatory details, Charges etc. Please note, this is for education purpose only. Please refer to the Privacy policy of MCA here
About Ministry of Corporate Affairs(MCA)
If you are a Chartered Accountant(CA) or running a private/publicly listed company in India, I am sure, you are aware of Ministry of Corporate Affairs(MCA). The Ministry of Corporate Affairs maintains a database of companies registered under the Companies Act. This contains information about companies, director information, financials, registration number commonly referred to Company Identification Number(CIN) among others.
Any registered company would have a unique Company Identification Number, commonly referred to as CIN. In order to obtain the company information, you would do the following:
- Visit Ministry of Corporate Affairs(MCA) website
- Click MCA Services -> View Company / LLP Master Data which takes you this link
- In the Company/LLP Master Data, you would enter the CIN of the company that you are searching followed by the captcha, and then enter
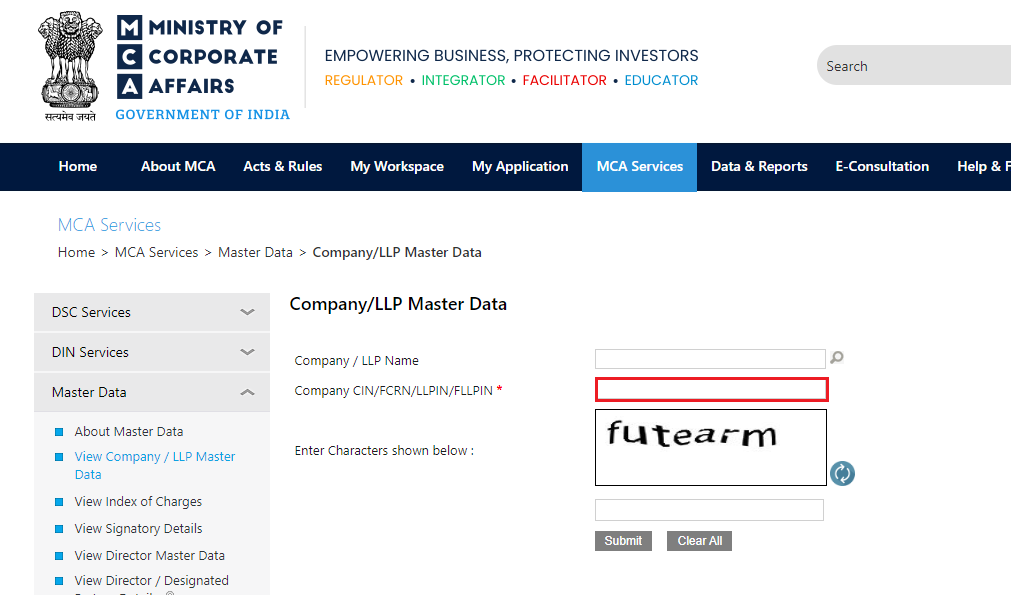
Any company which includes private, public or LLPs would display the information like:
- Company Name
- Date of Incorporation
- Company Type (public or private)
- Registered address
- etc
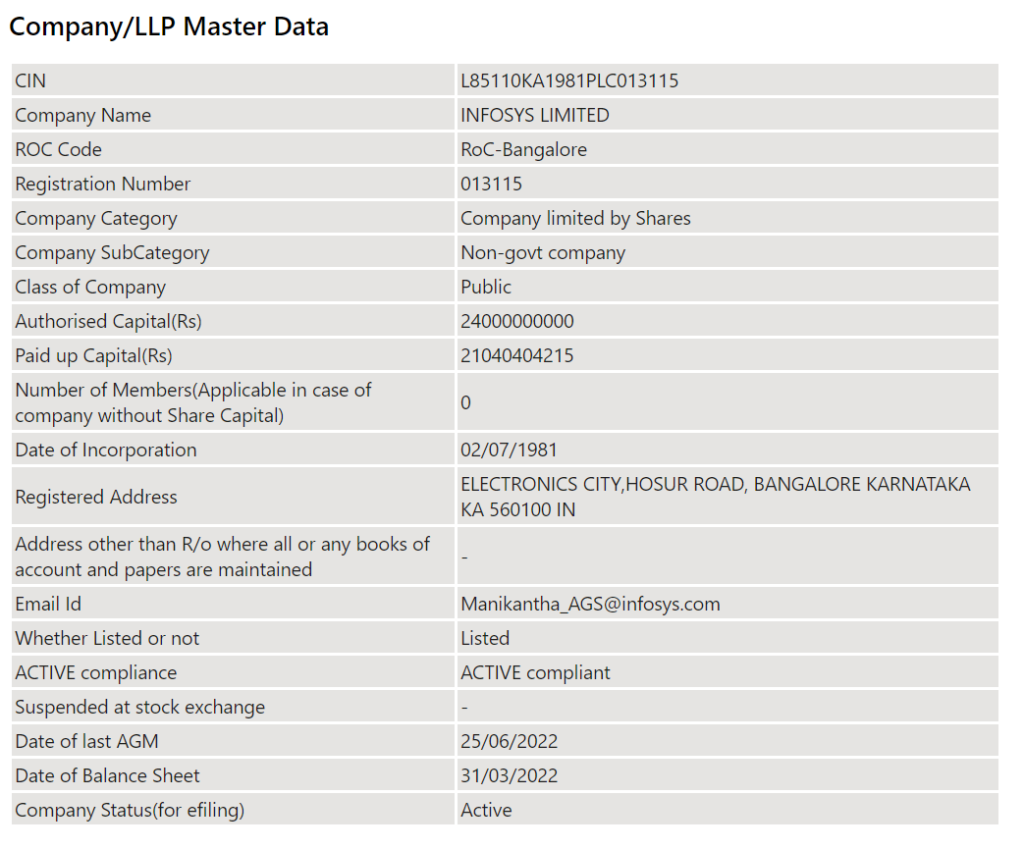
Python code to fetch company information
The below code snippet extracts the complete company information as list. Notice the URL https://www.mca.gov.in/mcafoportal/showdirectorMasterData.do. You need to send a POST request where companyid
is the CIN of the company.
I have noticed, that sometimes, the request sent via the application gets blocked when there are unusually high number of requests sent.
#---------------------------------------------------------------
# Fetch Company Information for the given CIN
#---------------------------------------------------------------
import pandas as pd
import requests
import html5lib
from bs4 import BeautifulSoup
def get_header():
headers = {}
headers['Origin'] = r'https://www.mca.gov.in'
headers['Referer'] = r'https://www.mca.gov.in/mcafoportal/showdirectorMasterData.do'
headers['Connection'] = r'keep-alive'
headers['Accept-Encoding'] = r'gzip, deflate, br'
headers['User-Agent'] = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/111.0.0.0 Safari/537.36'
return headers
def fetch_company_details(cin):
mca_url = r"https://www.mca.gov.in/mcafoportal/companyMasterDataPopup.do"
company_data = []
headers = get_header()
param = {'companyid': cin}
response = requests.post(mca_url, headers=headers, data=param, timeout=10)
soup = BeautifulSoup(response.content, 'html.parser')
tbl = soup.find("table",{"id":"resultsTab2"})
df = pd.read_html(str(tbl))[0]
df.columns = ['Attribute', 'Value']
for index, row in df.iterrows():
temp = {}
temp['Attribute'] = row['Attribute']
temp['Value'] = row['Value']
company_data.append(temp)
return company_data
if __name__ == '__main__':
cin = 'U74920DL2005PTC140111' #Sample CIN
company_data = fetch_company_details(cin)
print(company_data)
print("Done")
I hope you find this article helpful. The complete code to programmatically obtain the company information can be downloaded from my GitHub account here: https://github.com/kirancshet/MCA-CompanyInfo
1,392 total views, 4 views today