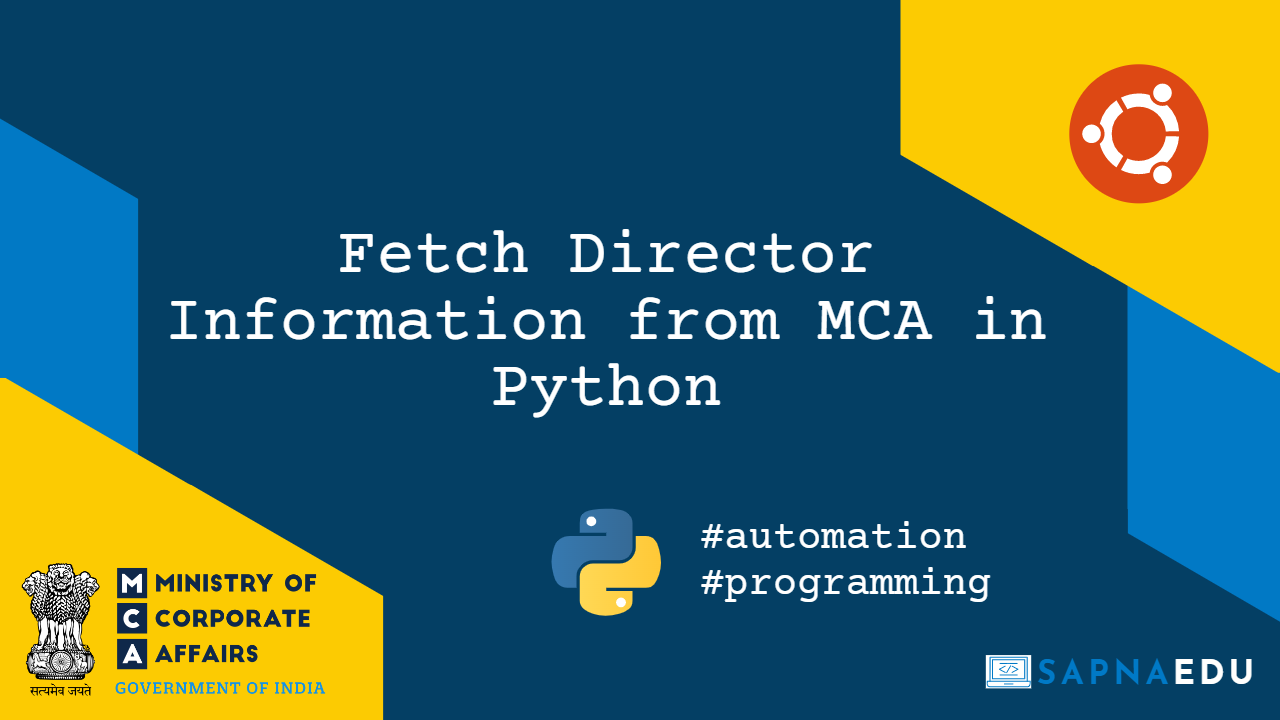
In this article, we will explore how to fetch Director Information from MCA in Python. This is a follow-up to my previous article on How to fetch Company Information from MCA in Python?
This article is part of a series of articles, where we explore automating MCA website typically used to obtain information like Director details, Company information, Signatory details, Charges etc. Please note, this is for education purpose only. Please refer to the Privacy policy of MCA here
About Ministry of Corporate Affairs(MCA)
If you are a Chartered Accountant(CA) or running a private/publicly listed company in India, I am sure, you are aware of Ministry of Corporate Affairs(MCA). The Ministry of Corporate Affairs maintains a database of companies registered under the Companies Act. This contains information about companies, directors, company financials among others. Each director has a unique 8-digit number called Director Information Number, commonly referred to as DIN.
In order to obtain the director information, you would do the following on the MCA portal:
- Visit Ministry of Corporate Affairs(MCA) website
- Click MCA Services -> View Director Master Data which takes you this link
- In the Director Master Data, you would enter the DIN of the director that you are searching followed by the captcha, and then enter
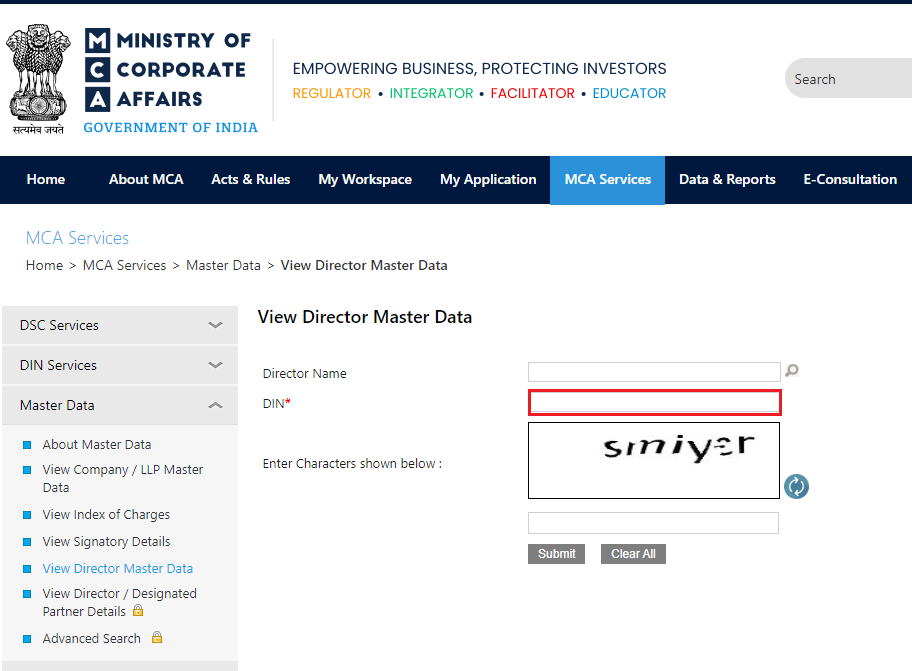
The director details include:
- Director Name
- List of companies including CIN details where he/she is a active director or was a director
- List of LLP details where he/she is a active director or was a director
- Start date of directorship
- End date of directorship ( if the director has resigned)
- etc
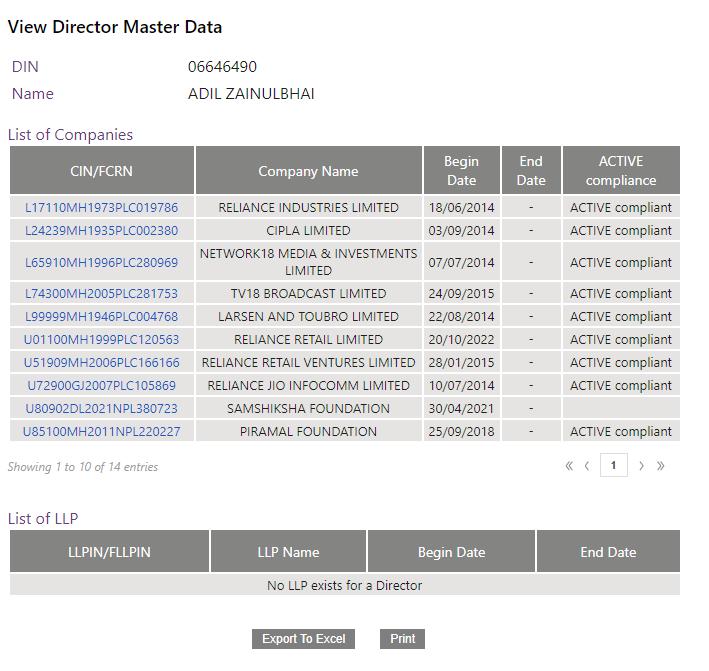
Python code to fetch director information
The below code snippet extracts the complete director information including the company details where he/she is a director. Notice the URL https://www.mca.gov.in/mcafoportal/directorMasterDataPopup.do. You need to send a POST request where din
is the DIN of the director.
I have noticed, that sometimes, the request sent via the application gets blocked when there are unusually high number of requests sent.
#-----------------------------------------------------------
# Fetch Director Information from MCA
#-----------------------------------------------------------
import pandas as pd
import requests
from bs4 import BeautifulSoup
def get_header():
headers = {}
headers['Origin'] = r'https://www.mca.gov.in'
headers['Referer'] = r'https://www.mca.gov.in/mcafoportal/showdirectorMasterData.do'
headers['Connection'] = r'keep-alive'
headers['Accept-Encoding'] = r'gzip, deflate, br'
headers['User-Agent'] = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/111.0.0.0 Safari/537.36'
return headers
def fetch_director_details(din):
director_details = {}
mca_url = r"https://www.mca.gov.in/mcafoportal/directorMasterDataPopup.do"
headers = get_header()
param = {'din': din}
response = requests.post(mca_url, headers=headers, data=param, timeout=10)
soup = BeautifulSoup(response.content, 'html.parser')
tbl = soup.find("table",{"id":"resultsTab2"})
df = pd.read_html(str(tbl))[0]
director_details['director_name'] = df.iloc[1,1]
tbl = soup.find("table",{"id":"resultsTab3"})
df = pd.read_html(str(tbl))[0]
director_details['company_details'] = df.to_dict('records')
return director_details
if __name__ == '__main__':
din = '06646490'
director_details = fetch_director_details(din)
print(director_details)
print("Done")
I hope you find this article helpful. The complete code to programmatically obtain the company information can be downloaded from my GitHub account here: https://github.com/kirancshet/MCA-CompanyInfo
1,318 total views, 2 views today