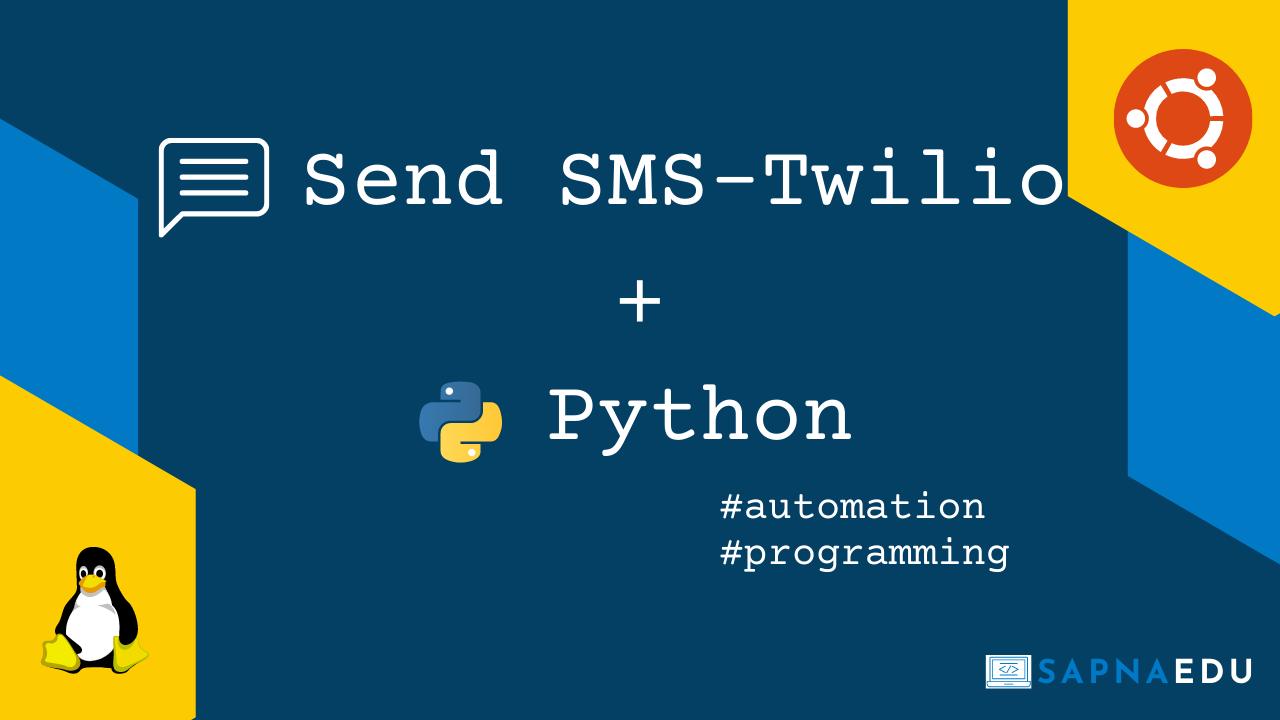
In this article, we will learn how to send SMS from your Python application. This is part of a series of article which I intend to cover on automating boring stuff.
You often come across a number of situation, where you need to send SMS to the user from your application. A typical case can be:
- To send verification code or One-Time Password(OTP) as part of Multi-Factor Authentication.
- Send Notification once the payment is received
- To send promotional messages as part of any campaign.
In order to send the SMS via an application, we need to rely on SMS Gateway provider. There are a number of SMS Gateway provider like:
- Twilio SMS
- Nexmo SMS
- ClickSend
- TeleSign
In this article, we will explore Twilio SMS Gateway to send SMS via Python application.
Step 1: Signup for a Twilio account here and login to your Twilio console. In the console, you will notice Account Info as shown below:
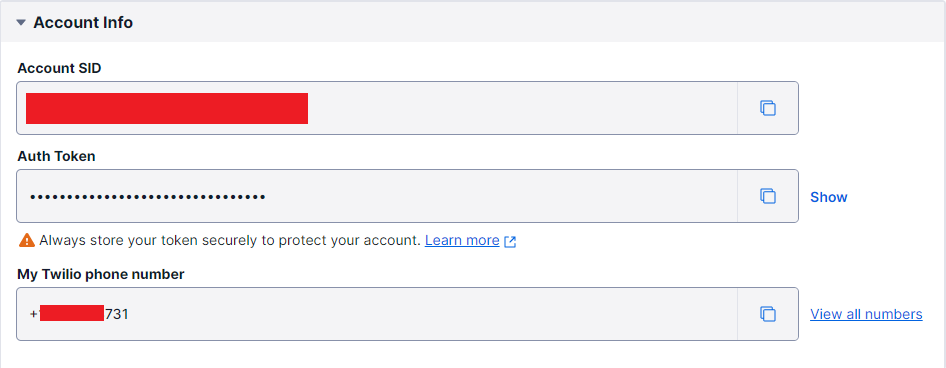
Now, copy the Account SID, Account Token and Phone number which we will be using in the Python application.
Step 2: Prerequisites
We will be using Twilio library to send the SMS. Install the twilio library via pip using the command:
pip3 install twilio
Once the above configuration is done, you are all set to send the SMS. If you have a trial account, you can only send the SMS to the verified mobile number. However, you can activate your account by charging your Twilio account which allows you to send the SMS to any number.
All the numbers should be formated in E.164 format which mandates the format for phone numbers into an internationally recognized format. In E.164 format, the mobile number would follow the format:
+[Country Code][Mobile Number]
Step 2: Python Code to send SMS
Here is the complete code snippet to send the SMS message to a destination mobile number
def send_sms_message(self, message, to_number):
ret = {}
ret['success'] = False
try:
client = Client(self.account_sid, self.auth_token)
param = {}
param['to'] = self.sanitize_mobile_number(to_number)
param['from_'] = self.from_number
param['body'] = message
response = client.messages.create(**param)
if hasattr(response, 'sid'):
ret['success'] = True
ret['sid'] = response.sid
except Exception as e:
print(str(e))
return ret
I hope you find this article helpful. The complete code to send SMS via Twilio can be downloaded from my GitHub account: https://github.com/kirancshet/SendSMS-Python
As an exercise, can you explore the following:
- Add Multiple recipients
- Personalize the SMS message by adding their first name in the SMS message
538 total views, 2 views today