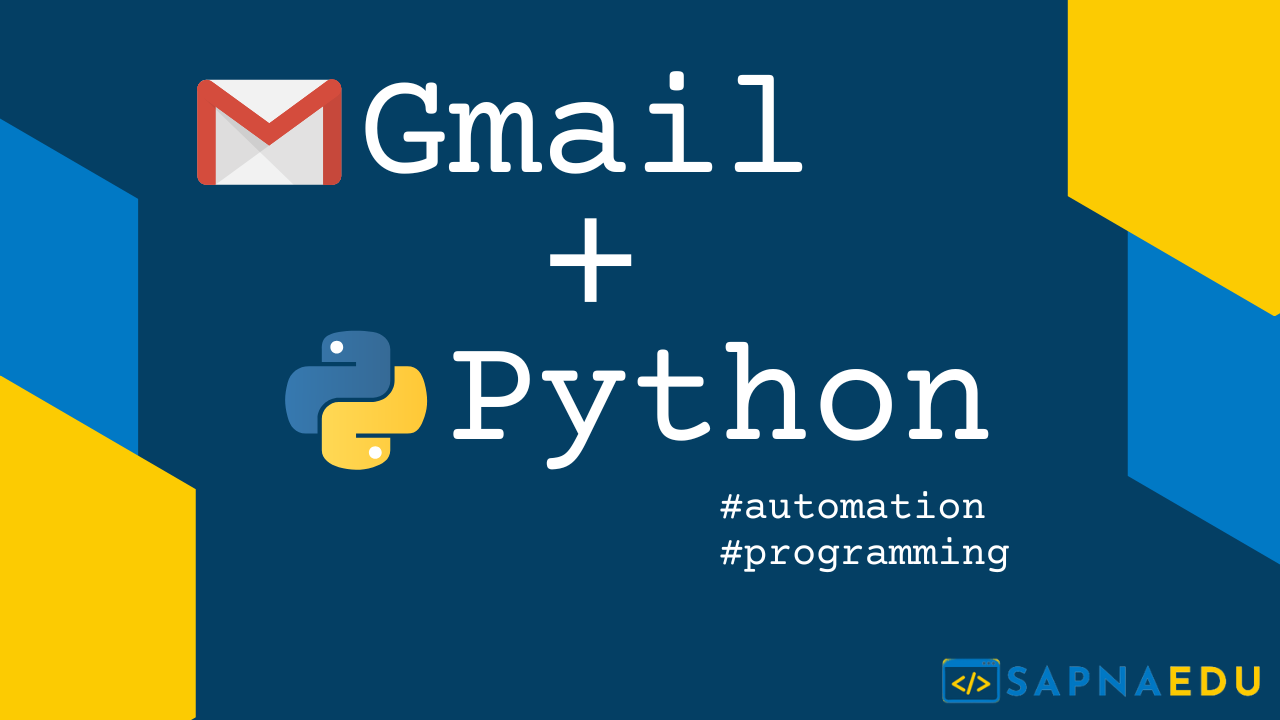
Just to give a background, you often come across a number of situation, where you need to send email from your application along with multiple file attachments. These email typically consists of daily/monthly reports/invoices that needs to be sent via the application. Instead of sending the email manually, we can automate the entire process where we can send Email with multiple attachments from your Gmail account in Python.
At the end of this article, you will know how to send email from your Gmail account along with multiple attachments in Python As a simple case study, let us send the email along with an Excel file and PDF attachment in the email.
This article is a follow-up to my earlier article on how to send email from your Gmail account in Python. If have not read it, you can read it here: https://sapnaedu.com/how-to-send-email-from-your-gmail-account/. This is part of a series of article which I intend to cover on automating boring stuff.
Gmail Security Setting
Step 1: I would assume that you have configured the security settings as explained here from your Gmail account and you have obtained app password.
Step 2: Python code to send Email from your Gmail account
We will be using smtplib
module to send email via SMTP (Simple Mail Transfer Protocol). You can use the following code to login to Outlook.
server = smtplib.SMTP(smtp.gmail.com, 587)
context = ssl.create_default_context()
server.starttls(context=context) # Secure the connection
server.login(self.username, self.password) # Use your Gmail username and app password here
Step 3: Prepare the Email message
Here, we are using MIME module to prepare the email message. We can send the email as a plain text or HTML. In this tutorial, let us consider sending a very simple email having HTML text.
msg = MIMEMultipart()
msg['From'] = self.username
msg['To'] = recipient_email_address # Single Email address
msg['Subject'] = "Test Email with multiple attachment"
msg.attach(MIMEText(email_message, 'html'))
I am assuming that, the recipient email address is a single email account. We can also send email to multiple recipients in which case, each email address needs to be separated by “,”.
For instance, if you need to send email to test1@email.com, test2@email.com, test3@email.com msg object would look like:
msg['To'] = "test1@email.com, test2@email.com, test3@email.com" # Multiple Email address
Step 4: Attach multiple files
Suppose you want to attach 2 files while sending the email. This can be using MIME package in Python
for ind_file in attachment_lst:
part = MIMEBase('application', "octet-stream")
part.set_payload(open(ind_file, "rb").read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', 'attachment; filename="%s"' % os.path.basename(ind_file))
msg.attach(part)
Step 5: The final step is to send the email
server.sendmail(self.username, recipient_email_address, msg.as_string())
Download:
The complete code to send the email from your Outlook account can be downloaded from my GitHub account here: https://github.com/kirancshet/SendEmail-Attachments
As an exercise, can you explore the following:
- Send Email to multiple recipients
- Add CC to your email
- Add BCC to your email
I hope you find this article helpful.
795 total views, 3 views today