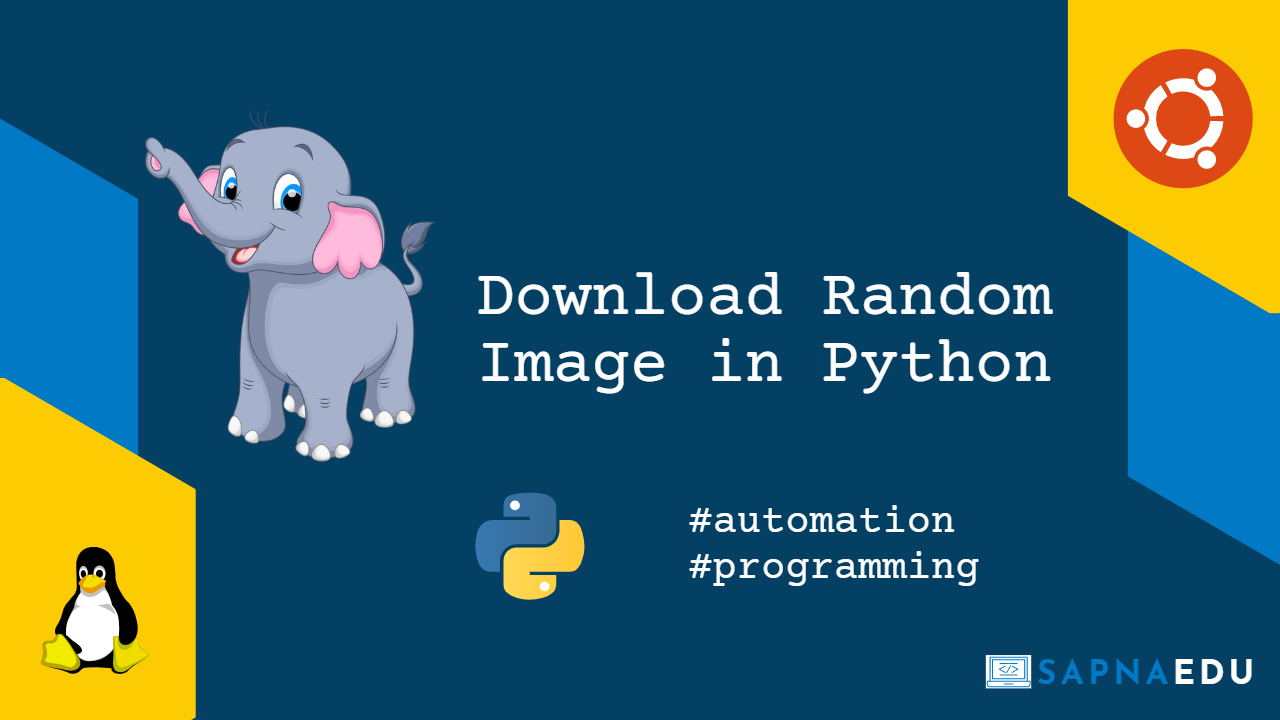
Download Random Image in Python
We often come across a number of situation wherein, we need to use some random images in our web application. Often we end up using some random image off the internet or downloading from sites like Shutterstock or Pexel manually and edit/crop the image and using it in our application.
In this article, we will explore how to download random image with a given specification like width, height, image type etc. As a use case, we will try to download 100 random image with the given specification:
- Image width – 400 pixel
- Image height – 300 pixel
- Image type – JPEG
- Grayscale image
There are a number of sites where random images can be downloaded programmatically. Please refer to lorem.space or picsum.photos for more information. In this article, we will explore picsum.photos for downloading images.
Step 1: Create the download URL
Depending on the configuration of the image, the URL to download the image would look something like below:
https://picsum.photos/<Image_Width>/<Image_Height>.<Image_Extension>?grayscale&blur
So, for instance, if you wish to download 400×300 jpeg image, the URL would be:
https://picsum.photos/400/300.jpg
If you would like a black & white image, the URL would be, https://picsum.photos/400/300.jpg?grayscale
Similarly, for a blur image, the URL would be, https://picsum.photos/400/300.jpg?blur
If you need a square image, you do-not have to specify both the image height and image width. The URL would be becomes: https://picsum.photos/400.png?blur&grayscale
Step 2: Python code to download image
The below code snippet downloads a single image and saves the image in the images folder.
import os
import requests
from urllib.parse import urlencode
from random import randint
#-----------------------------------------
# Download image from picsum.photos
#-----------------------------------------
def download_random_image(image_width, image_height):
extension = 'jpg' # png webp
gray_scale = False
blur_image = False
data = {}
if gray_scale:
data['grayscale'] = None
if blur_image:
data['blur'] = None # Add the blur level from 1 to 10
url = f"https://picsum.photos/{image_width}/{image_height}.{extension}?{urlencode(data)}"
filename = f"{randint(100_000,999_999)}.{extension}"
image_path = f"{os.getcwd()}/images/{filename}"
response = requests.get(url)
with open(image_path, mode='wb') as fp:
fp.write(response.content)
return True
download_random_image(400, 300)
That’s about it. Now, if you want to download let’s say 100 random images, simply call the method download_random_image
() in a for loop as shown below:
for i in range(0, 100, 1):
download_random_image(400, 300)
Just a note, I have not considered performance optimization. Please refer to the article here which talks of of performance optimization via asyncio/aiohttp. I hope, you find this useful.
The complete code to download 100 random images can be downloaded from my GitHub account here: https://github.com/kirancshet/Download-Random-Image
As an exercise, can you explore the following:
- Download 5 square black & white images in png format and send it as an attachment via Gmail. Please refer to my article here: How to send email from your Gmail account with multiple attachments in Python?
- Download 5 square black & white images in png format and create a zip file comprising of all the download images. Please refer to my article here: How to create a Zip file in Python?
332 total views, 2 views today