
Fetch reatime market price of a crypto currency from CoinGecko
Over the next few topics on automation, we will understand algorithmic cryptocurrency trading and explore some of the top crypto-tracking websites and their APIs. In this article, we will explore how to fetch real-time market price of cryptocurrency via CoinGecko in Python.
Why CoinGecko?
CoinGecko is a top crypo-tracking websites which provides real-time trading information tracking over 12,000 cryptocurrencies across more than 450+ exchanges. The CoinGecko provides REST API which provides a lot of information related to cryptocurrency like:
- Real-time market price
- Coin Information
- Historic data
- OHLC price
- Exchange information
CoinGecko is completely free with some restriction on the number of API calls at 10-50 requests per minute. However, there are premium plans available as well which offers additional benefits on the rate limits additional exchange support etc. If you are interested in the premium plans, you can subscribe here.
So, head over to CoinGecko and signup for a developer account. For the free plan, you donot need the API Key, however, if you are using any of the premium plans, you need to use API Key in your application.
With this background, let’s jump right into fetch the real-time price of few cryptocurrencies like BitCoin, Ethereum, Ripple etc.
CoinGecko API Endpoints
Depending on whether you are using a free developer account or a premium account, the API endpoint also changes.
#Premium Account API URL
API_URL = r"https://pro-api.coingecko.com/api/v3/"
# Free Account API URL
API_URL = r"https://api.coingecko.com/api/v3/"
Using API Keys (applicable only for premium accounts)
As mentioned earlier, you need to pass the API Key in every REST API call. This can be done in one of two ways:
- Via a custom header named x_cg_pro_api_key
- Via a query string parameter named x_cg_pro_api_key
Fetch the current market price of Bitcoin
Let’s say, we want to fetch the current market price of Bitcoin in US dollars. The current rate can be obtained using /simple/price
API endpoint.
import requests
API_URL = r"https://pro-api.coingecko.com/api/v3/" #Premium Account API URL
API_URL = r"https://api.coingecko.com/api/v3/" # Free Account API URL
def get_current_price(token:str, currency:str):
url = f"{API_URL}/simple/price"
header = {}
#header['x_cg_pro_api_key'] = '<API_KEY>' #Applicable only for premium account
header['Content-Type'] = 'application/json'
data = {'ids': token, 'vs_currencies': currency}
response = requests.get(url, headers=header, params=data)
crypto_info = response.json()
#print(json.dumps(crypto_info, indent=4))
return crypto_info
crypto_info = get_current_price('bitcoin', 'usd') #currency should be lower case
crypto_price = crypto_info[token][currency]
print(crypto_price)
You will a JSON response as follows:
# JSON Response providing the real-time price of BitCoin in US-Dollars
{
"bitcoin": {
"usd": 16821.81
}
}
crypto_price = crypto_info["bitcoin"]["usd"]
Fetch the current market price of multiple cryptocurrencies
The /simple/price
API supports multiple currencies via single API call. For instance, in order to fetch the market price BitCoin, Ethereum, Ripple, we need to pass the comma separated ids of the token.
crypto_info = get_current_price('bitcoin,ethereum,ripple', 'usd')
print(crypto_info)
# JSON Response providing the real-time price of BitCoin in US-Dollars
{
"bitcoin": {
"usd": 16824.74
},
"ethereum": {
"usd": 1264.09
},
"ripple": {
"usd": 0.337578
}
}
bitcoin_price = crypto_info["bitcoin"]["usd"]
eth_price = crypto_info["ethereum"]["usd"]
xrp_price = crypto_info["ripple"]["usd"]
If you are not familiar with the id of the token, you can get the API id from the CoinGecko website. For instance, lets say you are not familiar with the API id of Ripple, then head over to CoinGecko website search for Ripple and click on the market information page. Under the Info section, you will find the API id of the token.
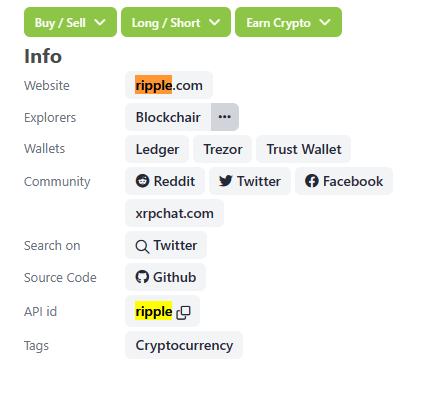
Current market price of multiple cryptocurrencies in multiple fiat currencies
In addition to supporting multiple cryptocurrencies, API also supports multiple fiat currencies. So, for instance, in order to fetch the market price BitCoin, Ethereum, Ripple, in US-Dollars, Euro and Indian Rupees and Canadian Dollars, we need to pass the comma separated ids of the token and currencies as follows:
crypto_info = get_current_price('bitcoin,ethereum,ripple', 'usd,eur,inr,cad')
print(crypto_price)
# JSON Response providing the real-time price of BitCoin in US-Dollars
{
"bitcoin": {
"usd": 16828.73,
"eur": 15842.92,
"inr": 1384906,
"cad": 22625
},
"ethereum": {
"usd": 1264.09,
"eur": 1190.04,
"inr": 104027,
"cad": 1699.51
},
"ripple": {
"usd": 0.337663,
"eur": 0.317883,
"inr": 27.79,
"cad": 0.453972
}
}
bitcoin_price_usd = crypto_info["bitcoin"]["usd"]
bitcoin_price_eur = crypto_info["bitcoin"]["eur"]
bitcoin_price_inr = crypto_info["bitcoin"]["inr"]
bitcoin_price_cad = crypto_info["bitcoin"]["cad"]
I hope you find this article helpful. The complete code to fetch real-time crypto price from CoinGecko can be downloaded from my GitHub account here: https://github.com/kirancshet/Crypto-RealTime-CoinGecko
As an exercise, can you explore the following:
- Get real-time crypto price of Bitcoin in US-Dollars and send an SMS. Please refer to my article here: How to send SMS via Twilio in Python?
- Get real-time crypto price of Bitcoin, Ethereum in US-Dollars and Euro and send an SMS.
358 total views, 2 views today