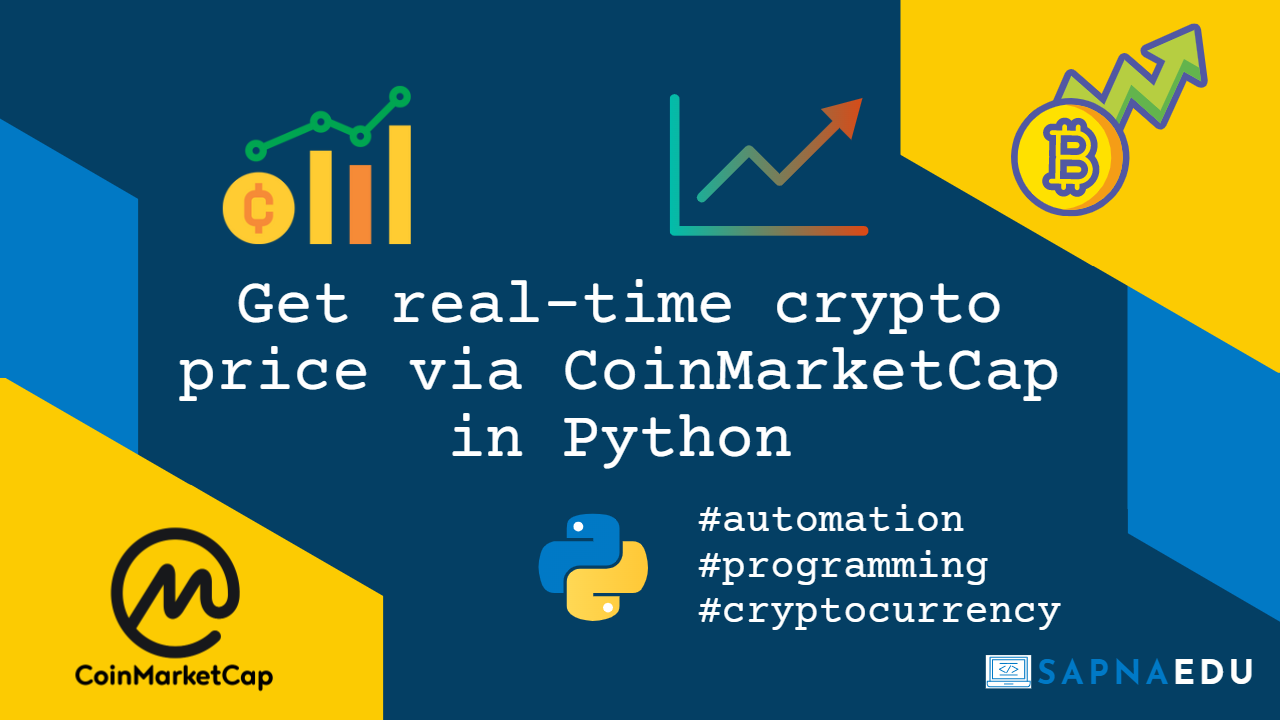
Fetch real-time market price of cyrptocurrency form CoinMarketCap in Python
In the previous article on How to fetch real-time market price of cryptocurrency from CoinGecko in Python?, we explored how to fetch real-time market price of cryptocurrency via CoinGecko in Python. In this article, we will understand how to fetch real-time market price of a cryptocurrency from CoinMarketCap in Python.
Why CoinMarketCap?
CoinMarketCap is a top crypo-tracking websites which provides real-time trading information tracking over 12,000 cryptocurrencies providing high-quality and accurate information The CoinMarketCap provides REST API which provides a lot of information related to cryptocurrency like:
- Real-time market price
- Coin Information
- Historic data
- OHLC price
- Exchange information
CoinMarketCap is completely free with some restriction on the number of API calls at 300 requests/day and API credit of 10,000 per month. However, there are premium plans available as well which offers additional benefits such as access to additional APIs and API credits etc. If you are interested in the premium plans, you can subscribe here.
So, head over to CoinMarketCap and signup for a developer account. Once your account is setup, you can get your API-Key from your developer dashboard https://pro.coinmarketcap.com/account
With this background, let’s jump right into fetch the real-time price of few cryptocurrencies like BitCoin, Ethereum, Ripple etc.
Fetch the current market price of Bitcoin
Let’s say, we want to fetch the current market price of Bitcoin in US dollars. The current rate can be obtained using /simple/price
API endpoint. The API endpoint for fetching the latest market quotes supports multiple crypto-currencies and multiple fiat currencies.
#CoinMarketCap API URL
API_URL = r"https://pro-api.coinmarketcap.com/v2/cryptocurrency/quotes/latest"
The complete code to fetch the current market price of Bitcoin in US dollars is shown below. Notice how the API-Key is passed in the header.
import requests
import json
#-----------------------------------------------------------
# Fetch Current market price of Crypto from CoinMarketCap
# Get your API from your developer account:
# https://pro.coinmarketcap.com/account
#-----------------------------------------------------------
def get_current_price(token:str, currency:str)->float:
url = r"https://pro-api.coinmarketcap.com/v2/cryptocurrency/quotes/latest"
header = {}
header['X-CMC_PRO_API_KEY'] = '<API_KEY>' #Get your API from your developer account
header['Content-Type'] = 'application/json'
data = {}
data['symbol'] = token
data['convert'] = currency
response = requests.get(url, headers=header, params=data)
crypto_info = response.json()
#print(json.dumps(crypto_info, indent=4))
return crypto_info
token = 'BTC'
currency = 'USD'
crypto_info = get_current_price(token, currency)
crypto_price = crypto_info['data'][token][0]['quote'][currency]['price']
print(crypto_price)
If the API-Key is valid, you should be receiving the JSON response as follows. Notice the credit count for the API call is 1. For a free developer account, you will have a hard-limit of 10,000 API credit counts. In addition the current market price, notice you can also get the following information in the same API call:
- Percentage change in last 24 hours
- Percentage change in last 7 days
- Percentage change in last 30 days
- Percentage change in last 60 days
- Percentage change in last 90 days
# JSON Response providing the real-time price of BitCoin in US-Dollars
{
"status": {
"timestamp": "2023-01-07T08:30:43.799Z",
"error_code": 0,
"error_message": null,
"elapsed": 24,
"credit_count": 1,
"notice": null
},
"data": {
"BTC": [
{
"id": 1,
"name": "Bitcoin",
"symbol": "BTC",
"slug": "bitcoin",
"num_market_pairs": 9922,
"date_added": "2013-04-28T00:00:00.000Z",
"tags": [
{
"slug": "paradigm-portfolio",
"name": "Paradigm Portfolio",
"category": "CATEGORY"
}...
],
"max_supply": 21000000,
"circulating_supply": 19254918,
"total_supply": 19254918,
"is_active": 1,
"platform": null,
"cmc_rank": 1,
"is_fiat": 0,
"self_reported_circulating_supply": null,
"self_reported_market_cap": null,
"tvl_ratio": null,
"last_updated": "2023-01-07T08:29:00.000Z",
"quote": {
"USD": {
"price": 16935.76451301602,
"volume_24h": 13529934710.628757,
"volume_change_24h": -2.9596,
"percent_change_1h": -0.06202264,
"percent_change_24h": 0.77329151,
"percent_change_7d": 2.25737861,
"percent_change_30d": 0.6259243,
"percent_change_60d": -14.12046876,
"percent_change_90d": -12.76180332,
"market_cap": 326096756965.4334,
"market_cap_dominance": 39.5761,
"fully_diluted_market_cap": 355651054773.34,
"tvl": null,
"last_updated": "2023-01-07T08:29:00.000Z"
}
}
}
]
}
}
crypto_price = crypto_price = crypto_info['data']['BTC'][0]['quote']['USD']['price']
Fetch the current market price of multiple cryptocurrencies
The API also supports multiple currencies via single API call. For instance, in order to fetch the market price BitCoin, Ethereum, Ripple, we need to pass the comma separated symbols of the token.
token = 'BTC,ETH,XRP'
currency = 'USD'
crypto_info = get_current_price(token, currency)
#print(json.dumps(crypto_info, indent=4))
bitcoin_price = crypto_info['data']['BTC'][0]['quote'][currency]['price']
ethereum_price = crypto_info['data']['ETH'][0]['quote'][currency]['price']
xrp_price = crypto_info['data']['XRP'][0]['quote'][currency]['price']
print(bitcoin_price)
print(ethereum_price)
print(xrp_price)
If you are not familiar with the symbol of the token, you can get the API symbol from the CoinMarketCap website. For instance, lets say you are not familiar with the API symbol of Ripple, then head over to CoinMarketCap website search for Ripple and click on the market information page. You can also pass the CoinMarketCap id or slugs which are unique to the cryptocurrency. Refer the API documentation for getting current market price via CoinMarketCap here which details additional parameters.
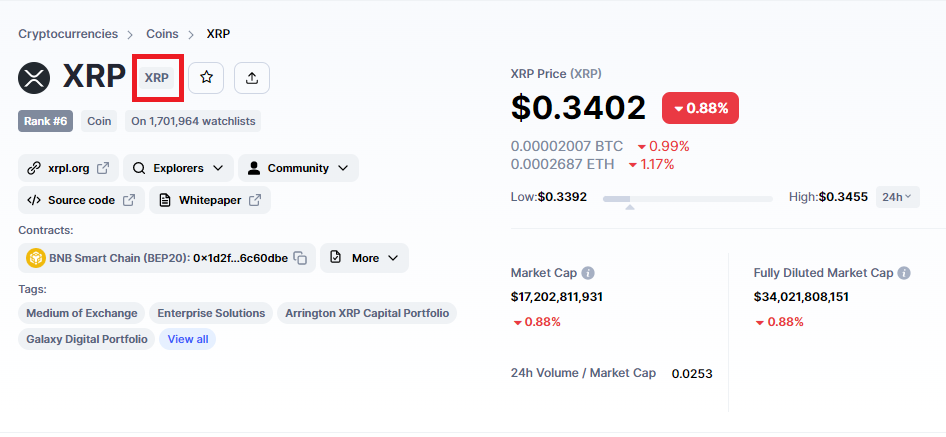
I hope you find this article helpful. The complete code to fetch real-time crypto price from CoinMarketCap can be downloaded from my GitHub account here: https://github.com/kirancshet/Crypto-RealTime-CoinMarketCap
As an exercise, can you explore the following:
- Get real-time crypto price of Bitcoin in US-Dollars and send an SMS. Please refer to my article here: How to send SMS via Twilio in Python?
- Get real-time crypto price of Bitcoin, Ethereum in US-Dollars and Euro and send an SMS.
810 total views, 2 views today