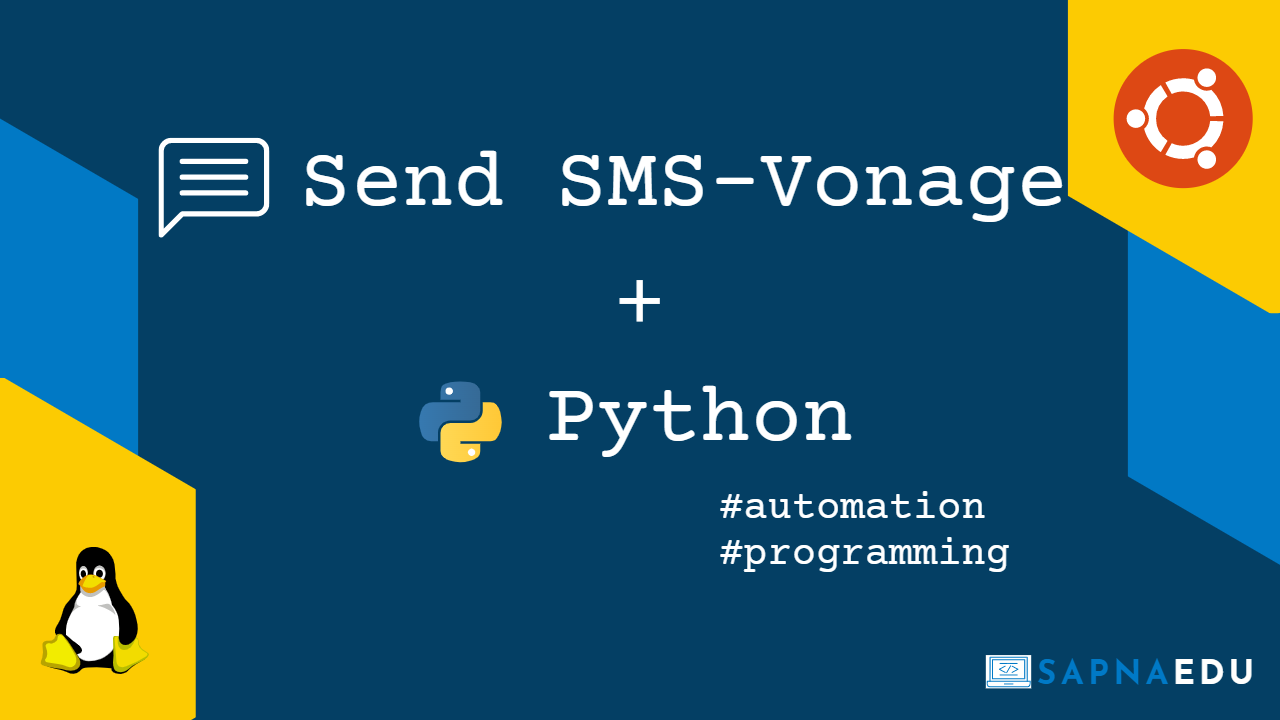
In this article, we will learn how to send SMS via Vonage SMS Gateway from your Python application. This is part of a series of article which I intend to cover on automating boring stuff.
In this article, we are going to be using Vonage, a very popular SMS Gateway for enterprise application.
You often come across a number of situation, where you need to send SMS to the user from your application. A typical case can be:
- To send verification code or One-Time Password(OTP) as part of Multi-Factor Authentication.
- Send Notification once the payment is received
- To send promotional messages as part of any campaign.
Step 1: Signup for a Vonage account here and login to your Vonage developer console. If you are on a trial account, you can send SMS only to the registered mobile number and also receive a credit of € 2.00 Under API settings, copy the API Key and API secret which we will be using in the Python application.
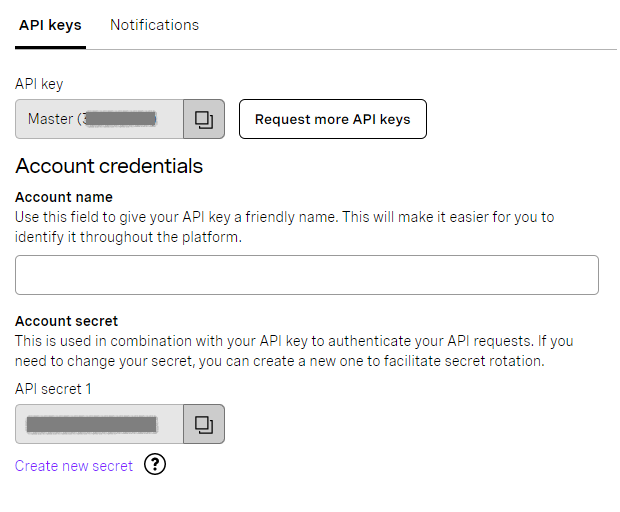
Step 2: Send your first SMS
In this article, we will be using Vonage SMS API to send the SMS. Please refer to the complete REST API documentation here. There is a useful guide on sending SMS using Python SDK from Vonage here
Once the above configuration is done, you are all set to send the SMS. If you have a trial account, you can only send the SMS to the verified mobile number. However, you can activate your account by charging your Vonage account which allows you to send the SMS to any number.
All the numbers should be formatted in E.164 format which mandates the format for phone numbers into an internationally recognized format. In E.164 format, the mobile number would follow the format:
[Country Code][Mobile Number]
Step 2: Python Code to send SMS
Here is the complete code snippet to send the SMS message to a destination mobile number
#---------------------------------------------------------------
# Send SMS using Nexmo
#---------------------------------------------------------------
def send_sms_message(self, message, to_number):
ret = {}
ret['success'] = False
try:
param = {}
param['api_key'] = self.api_key
param['api_secret'] = self.api_secret
param['from'] = self.from_number
param['text'] = message
param['to'] = self.sanitize_mobile_number(to_number)
param['callback'] = r'https://webhook.site/4251d8c3-be31-4e00-9dcb-298252348a02' #Callback URL
response = requests.get(self.base_url, params=param)
if response.status_code == 200:
json_response = response.json()
if 'messages' in json_response:
ret['message_id'] = json_response[0]['message-id']
ret['success'] = True
except Exception as e:
print(str(e))
return ret
I hope you find this article helpful. The complete code to send SMS via Vonage can be downloaded from my GitHub account: https://github.com/kirancshet/SendSMS-Nexmo-Python.
As an exercise, can you explore the following:
- Handle the SMS delivery to verify if the SMS is delivered
- Add Multiple recipients
- Personalize the SMS message by adding their first name in the SMS message
760 total views, 2 views today