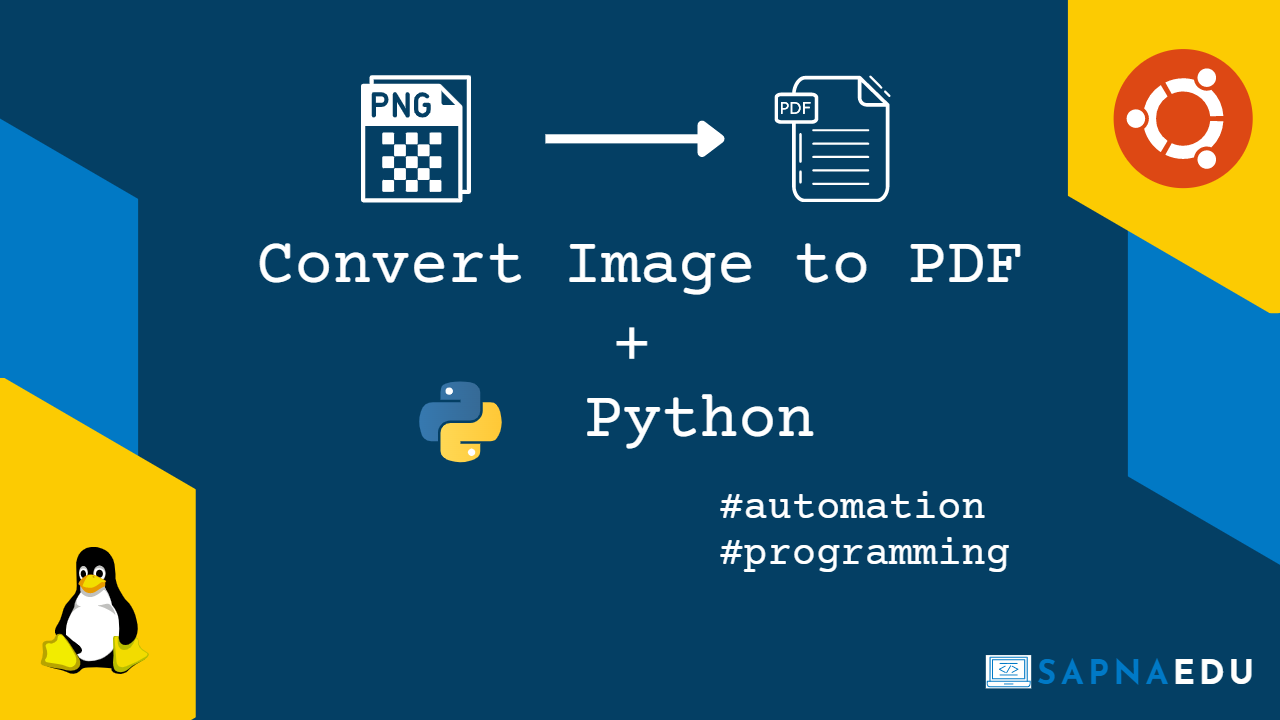
Convert Image to PDF in Python
In this tutorial, we will see how to convert an image into PDF. This is part of our continued series on automating boring stuff in Python.
At the end of this tutorial, you will learn how to convert an image( png, jpeg) into a PDF file using Python. To convert the image, we will be using Image processing library PIL(Python Image Library)
Step 1: Install the Python Image Library
Install the PIL library from the command prompt using the command:
pip install Pillow
Step 2: Convert the image to PDF
The image can be in png or jpg format. Suppose you want to convert a single image to PDF, the below code snippet takes the image as an input and generates the PDF file.
import os
from PIL import Image
#------------------------------
# Convert image to PDF
#------------------------------
def convert_image_pdf(image_path):
pdf_file = f"{os.getcwd()}/output/test.pdf"
img = Image.open(image_path)
im_1 = img.convert('RGB')
im_1.save(pdf_file)
return pdf_file
image_path = f"{os.getcwd()}/input/test_image.jpg"
pdf_file = convert_image_pdf(image_path)
print(pdf_file)
Step 3: Convert multiple images to PDF
We can also convert multiple images into a single PDF file. The below code snippet takes multiple images as an input and generates a single PDF file comprising all the images as separate page.
import os
from PIL import Image
#------------------------------------------
# Convert multiple images into single PDF
#------------------------------------------
def convert_multiple_images_pdf(self, file_list:list)->str:
pdf_file = f"{os.getcwd()}/output/combined.pdf"
img_list = [Image.open(image_path) for image_path in file_list]
img_list[0].save(pdf_file, "PDF", optimize=True, save_all=True, append_images=img_list[1:])
return pdf_file
I hope you found this article useful. You can download the complete source code from my GitHub account here: https://github.com/kirancshet/Convert-Image-PDF-Python
As an exercise, can you explore the following:
- Download 5 random images in Python and convert into 5 PDF files representing each image downloaded. You can refer to the article here
- Download 5 random images in Python and convert into a single PDF file and send it as an attachment via Gmail. You can refer to the article here: How to send email from your Gmail account with multiple attachments in Python?
388 total views, 2 views today