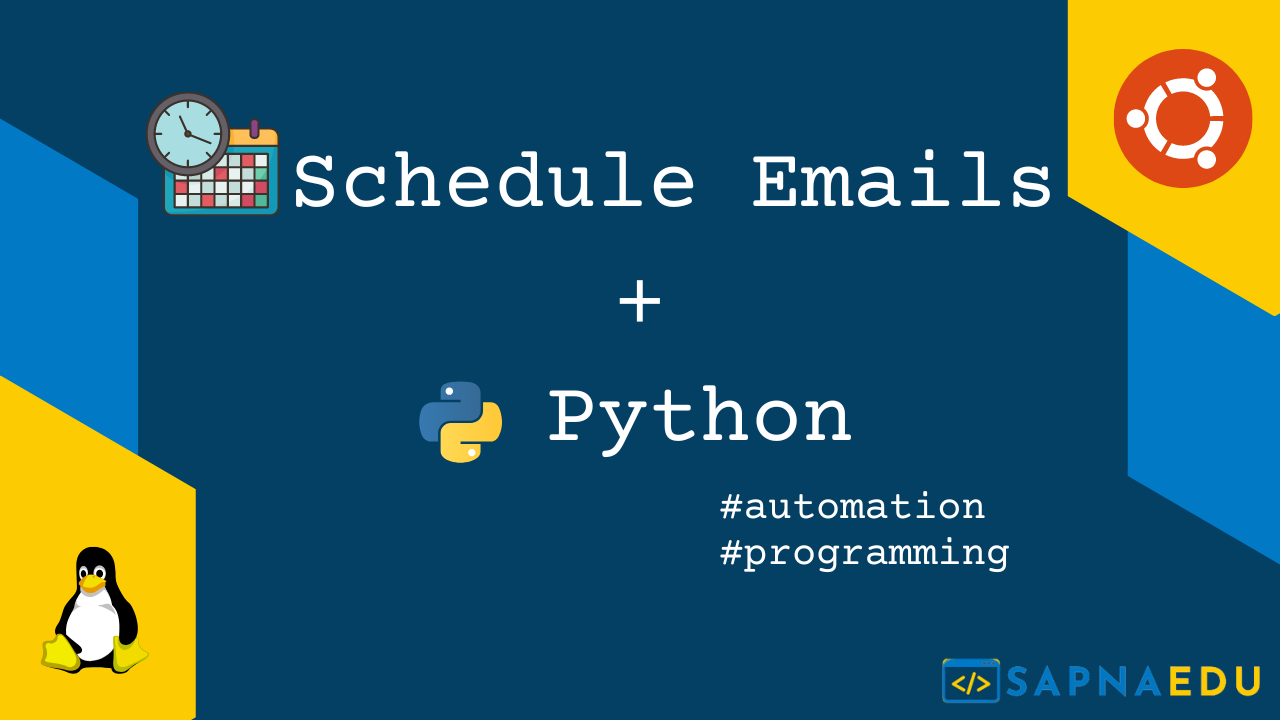
You often come across a number of instances, where we need to send an email at a specific time rather than immediately. For instance, birthday of your friends, anniversary, weekly financial report to your client to name a few. In principle, you can send the email manually every time, however, we can as well automate the entire process through a scheduler. In this article, we will explore how to schedule an email in Python.
For simplicity, we will use Gmail as the Email provider, the process remains the same others like Outlook. At the end of this article, you will learn on how to:
- Configure your Gmail account to send Email.
- Check if the user’s birthday is today?
- Send birthday greetings as an email.
- Schedule an email to send birthday greetings
Step 1: Configure your Gmail account to send Email
Configuring your Gmail account to send email via the application involve modifying security settings. There is a whole article on the entire configuration process here.
Step 2: Check if the user’s birthday is today
As a use-case for this article, let us assume that we need to send Birthday wishes to a number of our friends. On their birthday, the application should send a personalized email message with birthday wishes. Let us assume we have the following users:
# User's List as a list of dictionary consisting of their name, birthday and email
names = []
names.append({'birthday': '18-Oct', 'email': 'john@gmail.com', 'name': 'John'})
names.append({'birthday': '15-Oct', 'email': 'melissa@gmail.com', 'name': 'Melissa'})
names.append({'birthday': '26-Sep', 'email': 'sandra@gmail.com', 'name': 'Sandra'})
names.append({'birthday': '12-Jun', 'email': 'steve@gmail.com', 'name': 'Steve'})
names.append({'birthday': '03-Dec', 'email': 'bill@gmail.com', 'name': 'Bill'})
Notice that, all the birthdays on different dates. The idea is to check the birthday of all the users with the current date
def check_birthday_is_today(self, name):
success = False
try:
current_day = datetime.today().day
birthday = parse(name['birthday'])
if birthday.day == current_day:
success = True
except Exception as e:
print(str(e))
return success
If the date matches, we need to send a personalized email message. Let us add placeholders in the email message to make it personalized as follows:
message = '''
<p>Hi {name}, </p>
<p>I wish you a Very Happy Birthday.
May this year bring you greater success and achievements in your career
and happiness to cherish in the years to come.</p>
<img src="https://raw.githubusercontent.com/kirancshet/birthday-wishes-python/main/assets/birthday{rand_num}.png" width="600">
<p>Regards,<br/>
Sapnaedu</p>
'''
subject = "Happy Birthday {name}"
Step 3: Send birthday greetings as an email.
As we have the personalized email message for the user, the only step remaining is to send the email to the user. Here is the code snippet to send the email to the user using your Gmail account.
# Loop through all the names
for ind in names:
if self.check_birthday_is_today(ind):
ind['rand_num'] = randint(1,5)
email_subject = subject.format(**ind)
email_message = message.format(**ind)
obj = send_email.SendEmail()
obj.send_email(email_subject, ind['email'], email_message) #Send Email from your Gmail Account
Step 4: Schedule an email to send birthday greetings
The final step is to setup a scheduler, which runs the above script every day to check if the user’s birthday is today. In order to send the birthday, it is sufficient to run once every day. Let us run our python program once at 10AM.
I assume, we will be using a Linux system. In Linux, we can use cron
to create a scheduler. All the cron
job entries are found in /etc/crontab.
You can add an entry in the crontab file using the following command in the shell:
# Add a new entry in the crontab file
crontab -e
# Add the following line at the end of the file
0 10 * * * /user/local/bin/python3 /<path_to_python_script_folder>/send_birthday_wish.py
You can check if the above scheduler is running using the command crontab -l
I hope you find this article helpful. The complete code to schedule an email can be downloaded from my GitHub account here: https://github.com/kirancshet/birthday-wishes-python
As an exercise, can you explore the following:
- Add CC/BCC to your email
- Attach a simple document with the email
694 total views, 2 views today